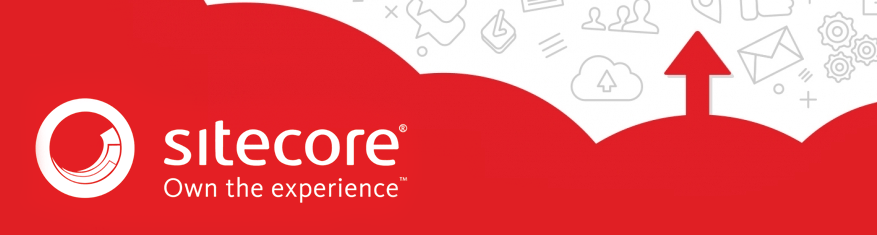
When an email campaign is dispatched, it moves through a number of states (Draft, Queuing, Sending, and Sent). If an error occurs during dispatch and the IIS server is interrupted, the email campaign is not moved to the Sent state. Instead, it is moved to the Paused state and the user must resume the email campaign manually.
As an alternate solution, we will create a task scheduler, which will scan all the emails and will return a list of paused email campaigns. Then it will resume paused dispatches.
At first, we are going to create a task to get a list of paused emails. We create a class called “ResumePausedEmail”, we will need “EcmDataProvider” and “ManagerRootService”. Initialize both in the constructor:
private readonly EcmDataProvider _dataProvider;
private readonly IManagerRootService _managerRootService;
public ResumePausedEmail() : this(ServiceLocator.ServiceProvider.GetService<EcmDataProvider>(), ServiceLocator.ServiceProvider.GetService<IManagerRootService>())
{
}
internal ResumePausedEmail([NotNull] EcmDataProvider dataProvider, [NotNull] IManagerRootService managerRootService)
{
_dataProvider = dataProvider;
_managerRootService = managerRootService;
}
The following code will return ManagerRoots:
List<ManagerRoot> managerRoots = _managerRootService.GetManagerRoots();
The following code will return Campaings which are “Paused” or “Queueing” status.
var options = new CampaignSearchOptions
{
ManagerRootId = managerRoot.Id,
StatusInclude = new[]
{
EcmTexts.Paused,
EcmTexts.Queuing
},
Page = new DataPage
{
Index = 0,
Size = 10
}
};
CampaignSearchResults campaigns = _dataProvider.SearchCampaigns(options);
To resume emails, we will need “SendingManagerFactory” and “ExmCampaignService”. Then loop through the email campaigns and resume one by one.
var sendingManagerFactory = ServiceLocator.ServiceProvider.GetService<ISendingManagerFactory>();
var campaignService = ServiceLocator.ServiceProvider.GetService<IExmCampaignService>();
foreach (EmailCampaignsData campaign in campaigns)
{
MessageItem emailCampaign = campaignService.GetMessageItem(campaign.MessageId);
ISendingManager sendingManager = sendingManagerFactory.GetAsyncSendingManager(emailCampaign);
sendingManager.ResumeMessageSending();
}
Now, we need to create a Task Scheduler. This has 2 parts, the first is to create Command, and the second is to create a Schedule. Task is located in “/sitecore/system/Tasks”.
Now, create a command and call your task (we have created above) from the command.
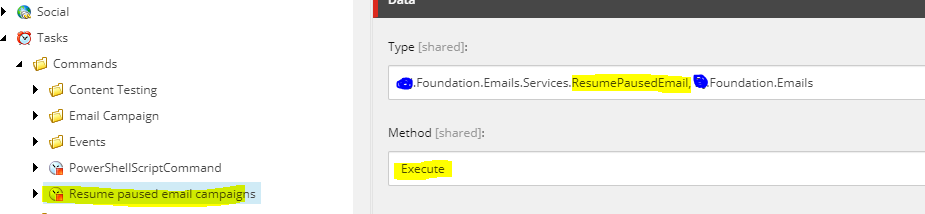
In the Schedule, first thing is to select the previously created command. Then we have to define Start date-time, End date-time, and interval.
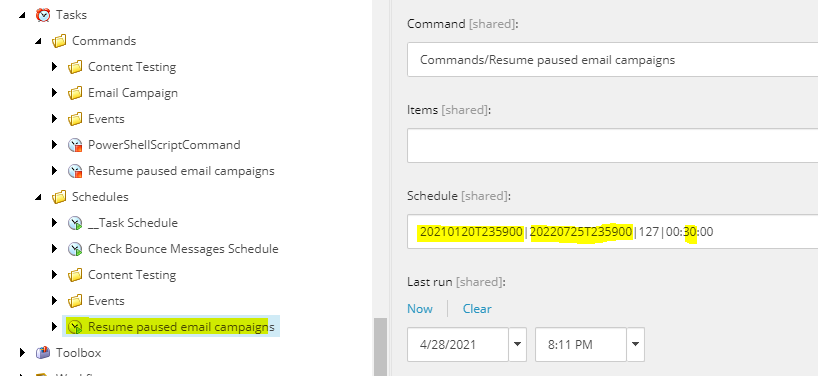
Now, the schduler is ready to resume pasued/ queueing emails.
Thanks
Munir