Recently we tried different Json rendering listing components. One of them was a SummaryComponent.
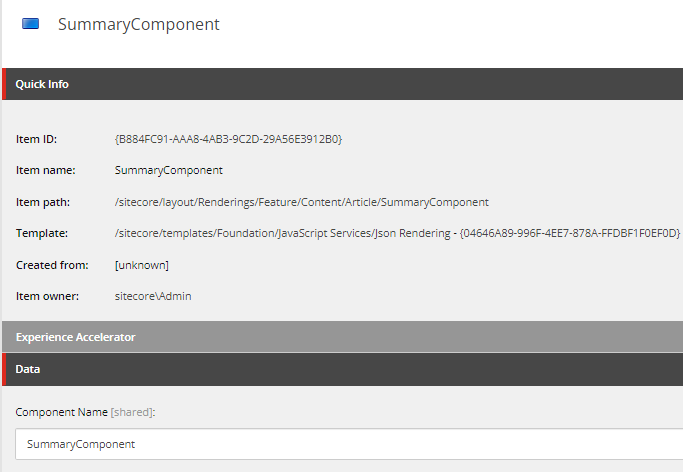
Set the LayoutService to Datasource Item Children Resolver.
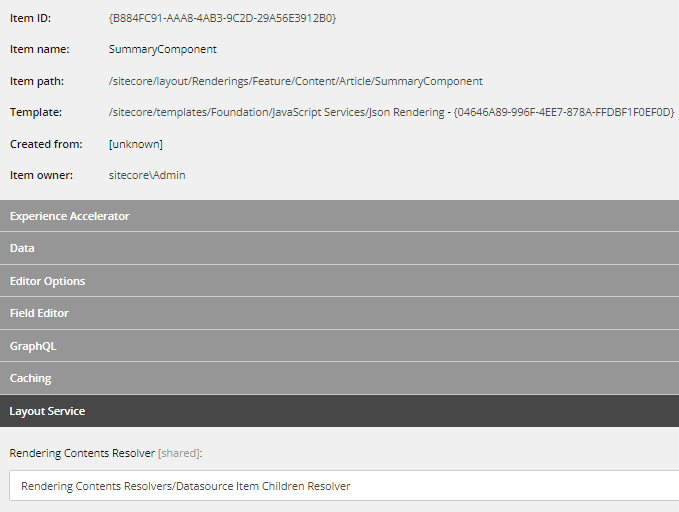
JSS component will look like as follows (we have a custom theme)
import {
RichText,
Field,
withDatasourceCheck,
ImageField,
Image,
} from '@sitecore-jss/sitecore-jss-nextjs';
import { styled } from '@mui/material/styles';
import { ComponentProps } from 'lib/component-props';
import { xxxText } from '@xxx/yyy.components';
import { xxTheme } from '@xxxx/yyy.theme';
import { SitecoreHeading } from '@fe-jss-nx/sitecore-ui';
type SummaryProps = {
id: string;
fields: {
SummaryTextField: Field<string>;
SummaryTextIconField: ImageField;
HeadingTextField: any;
};
};
type SummaryComponentProps = ComponentProps & {
fields: {
items: SummaryProps[];
};
};
const StyledMessagesListCard = styled('div', { name: 'MessagesListCard' })(
({
theme: {
xxx: { palette, spacing },
},
}: {
theme: xxxTheme;
}) => ({
backgroundColor: palette.neutral.background.medium,
padding: spacing(600),
width: '100%'
})
);
const StyledMessagesListItem = styled('div', { name: 'MessagesListItem' })(
({
theme: {
xxx: { spacing },
},
}: {
theme: xxxTheme;
}) => ({
display: 'flex',
alignItems: 'center',
gap: spacing(100),
marginBottom: spacing(100),
})
);
const SummaryComponent = ({ fields }: SummaryComponentProps): JSX.Element => (
<StyledMessagesListCard>
<SitecoreHeading.H2 fields={{HeadingTextField: fields.items[0].fields.HeadingTextField}} params={{}} />
<div className="sc-cards">{fields?.items?.map((card) => card && <Card {...card} />)}</div>
</StyledMessagesListCard>
);
const Card = ({ fields, id }: SummaryProps): JSX.Element => (
<div className="sc-card" key={id}>
<StyledMessagesListItem>
<Image field={fields.SummaryTextIconField} />
<xxxText size='bodySm' component='div'>
<RichText field={fields.SummaryTextField} tag="p" />
</xxxText>
</StyledMessagesListItem>
</div>
);
export default withDatasourceCheck()<SummaryComponentProps>(SummaryComponent);
For the listing item, we also need to create a Data template.

We will need a heading Data template.
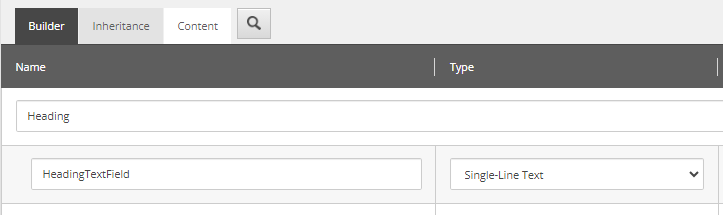
We will also need a SummaryComponent Data template as a parent.
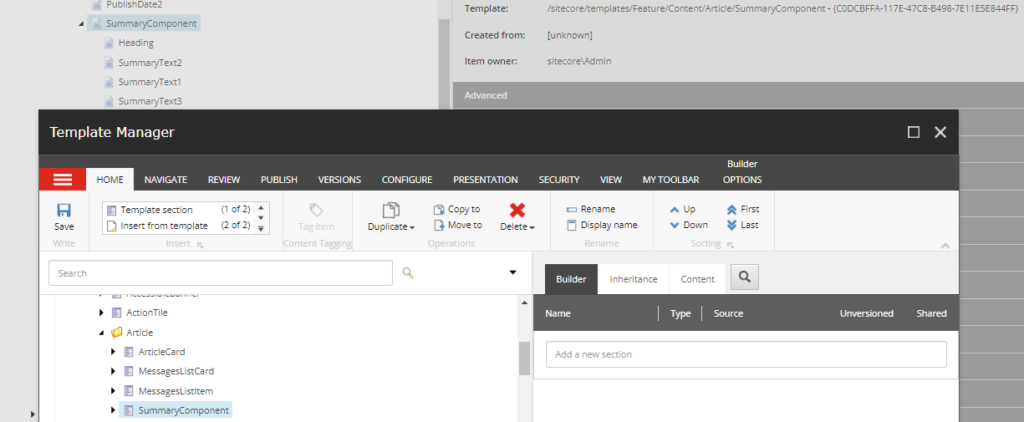
Finally, when we drop the component into a page and give the Datasource path it will look like the following.
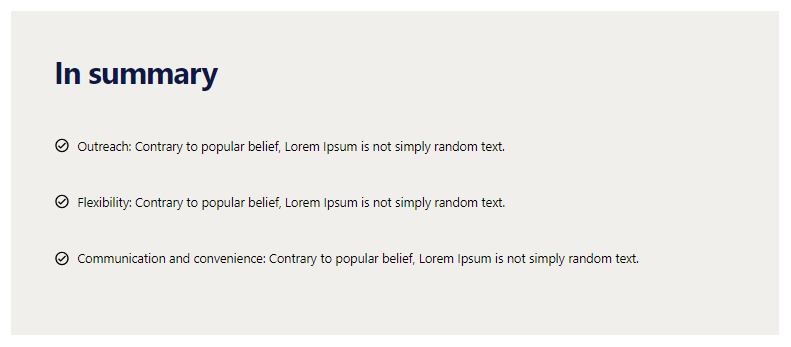
Happy Coding.